What is the difference between useState and useRef for react in a form?
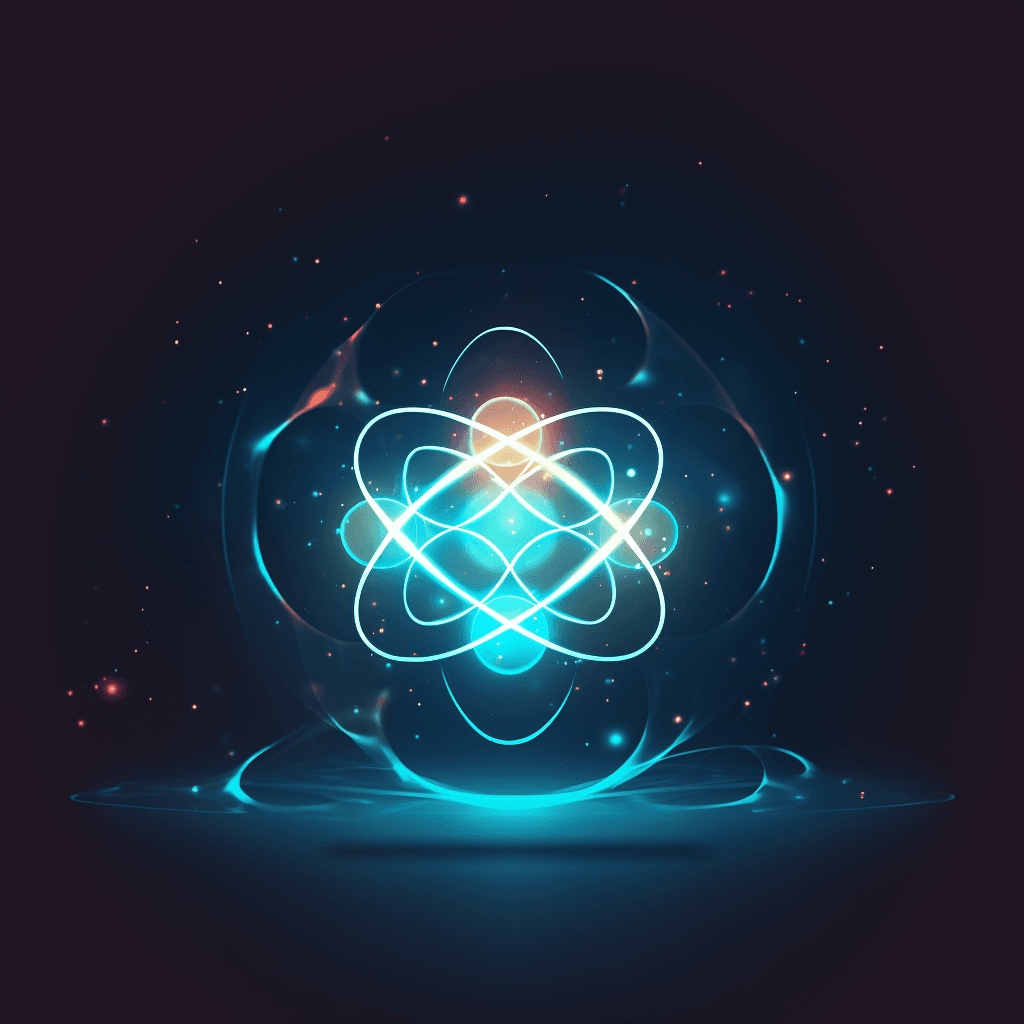
React hooks are used to add state to your component. They can be used in both functional components and classes, but they’re especially useful if you want to define state outside of the render method. In this post, we’ll go over two hooks that can help with this: useState and useRef. We’ll also look at how these work inside of a simple form so you can see how they’re different from each other when it comes time to use them in your own projects!
First, useState is a hook available to functional components that allows you to add state to your component. On the other hand, useRef is a hook used to set a ref on an element that you want to focus on when a component mounts.
The useState function returns an array, with the first element being the current state and the second being a function. The function receives two arguments: currentState and setState. You can update the state by calling this function:
const [title, setTitle] = useState("");
console.log(title)
// returns ''
setTitle('Blog Title')
// returns 'Blog Title'
Notice that the useState function returns an array with two values in it. The first value is the current state value, and the second value is a function that you can use to update the state (usually you will set this equal to a variable like setValue)
You can think of useState as a wrapper for the setTitle() function. You’re still using it to update your state, but you’re doing so via a helper function instead of using the raw setTitle() call directly.
It returns an array with two values in it:
-
The first value is the current state value, and
-
The second value is a function that you can use to update the state (usually you will set this equal to a variable like setValue).
Note that useState takes one argument, which is the initial state of our component. In this case, we are setting it equal to an empty string because we will be storing data about an input field(that does not have any value when the component first mounts). However, if we wanted to store data about something else with an initial value on it, we can pass something different into useState like so:
const [title, setTitle] = useState("This is my initial title");
-
useState is a hook available to functional components that allows you to add state to your component.
-
On the other hand, useRef is a hook used to set a ref on an element that you want to focus on when a component mounts.
Now that we know what useState does, let’s look at how to interact with this state in our component. Now that we know what useState does, let’s look at how to interact with this state on an input field in our component.
The first thing you’ll want to do is set a value for the input which is the initial state of the title. As the user types and changes we then want to use an onChange handler and setTitle to the event.target.value which triggers a re-render of the application:
import { useState } from "react";
const [title, setTitle] = useState("");
<label>Hotel title:</label>
<input
type="text"
required
value={title}
onChange={(e) => setTitle(e.target.value)}
/>
On the other hand useRef is a pointer to keep an eye on the input which is better for performance as it does not re-render the application and also is a cleaner syntax:
import { useRef } from "react";
const titleRef = useRef<HTMLInputElement>(null);
<label>Hotel title:</label>
<input type="text" required ref={titleRef} />
Conclusion
useState is a great way to handle state in your components, and it’s pretty easy to use. The useRef hook is also another way to track elements in a form also. If you want a more in-depth tutorial on this then please check my Youtube Video comparing both and refactoring from useState to useRef.
You can watch the video of this explanation here.
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!