What is the difference between mapStateToProps() and mapDispatchToProps() explain like i’m 5?
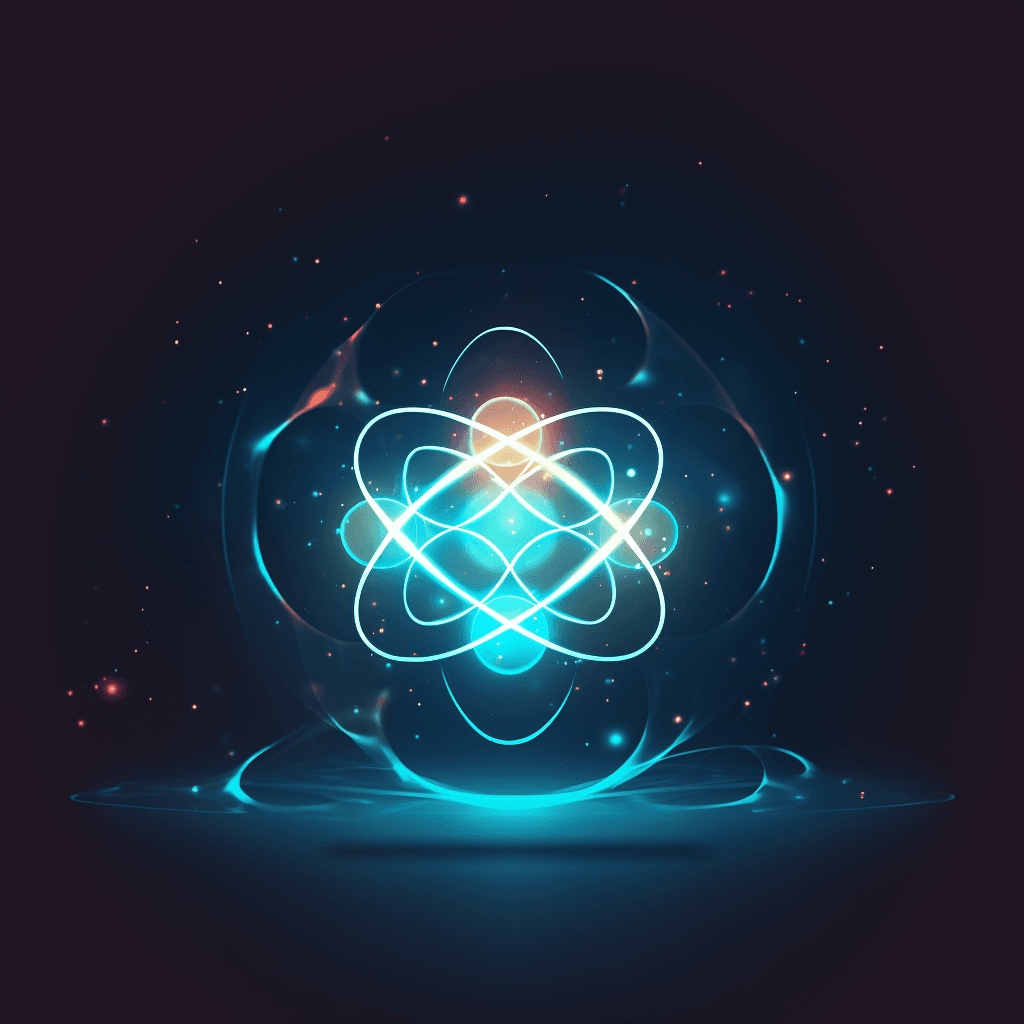
In Redux, we have something called a store that holds all the data of our application. mapStateToProps and mapDispatchToProps are two functions that allow our React components to interact with this store.
mapStateToProps helps us “read” data from the store. It takes the data from the store and maps it to the props of our component, so that we can use it in our component.
On the other hand, mapDispatchToProps helps us “write” data to the store. It maps action creators (which are functions that return an action object) to props of our component. When we call these functions in our component, they create an action object and send it to the store, which then updates the data in the store.
import { connect } from 'react-redux';
const MyComponent = ({ name, onClick }) => (
<div>
<p>My name is {name}.</p>
<button onClick={onClick}>Change Name</button>
</div>
);
const mapStateToProps = (state) => ({
name: state.name
});
const mapDispatchToProps = (dispatch) => ({
onClick: () => dispatch({ type: 'CHANGE_NAME', payload: 'John' })
});
export default connect(mapStateToProps, mapDispatchToProps)(MyComponent);
So, mapStateToProps helps us get data from the store, and mapDispatchToProps helps us change data in the store.
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!