What is GraphQL and how to fetch data with it in your frontend react application?
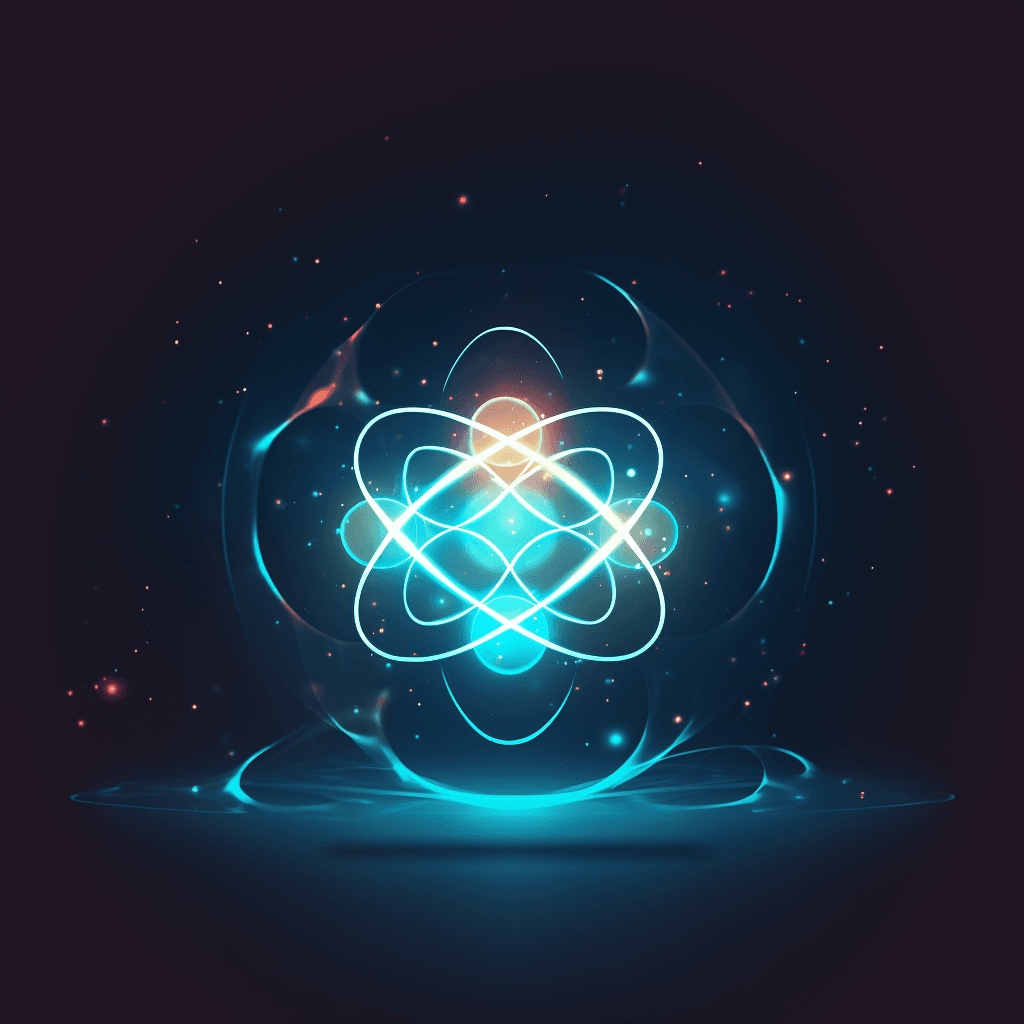
GraphQL is a data query language developed by Facebook. It lets you write queries to get your data from a server, instead of writing multiple REST API calls with different endpoints and parameters. In this tutorial, we will learn how to fetch data using GraphQL in our React application.
What is GraphQL?
GraphQL is a query language for APIs. It allows clients to specify exactly which data they need from the server and has built-in caching.
GraphQL is a type system for APIs, which means that it allows you to define your API schema in advance, and then you can use this schema when writing queries or mutations (additional request types) for your web application.
One benefit of this approach is that your GraphQL API will always be correct: if there’s an error in any part of the system, it’ll be caught at compile time instead of runtime.
GraphQL also supports introspection (a way to discover information about the state of the system), so you can find out how much data has been fetched by using its full-text search capabilities. Finally, GraphQL comes with its own runtime that can be used on both client-side (React) as well as server-side (Node).
Let’s look at the steps on how you can fetch GraphQL data in your react app.
Install Apollo Client and GraphQL with the following command:
npm i @apollo/client graphql
OR
yarn add @apollo/client graphql
Now let’s create a GraphQL folder and create two files
client.ts – This file creates the client and tells what endpoint we use to fetch the data. This gets passed into our ApolloProvider later on.
import { ApolloClient, HttpLink, InMemoryCache } from '@apollo/client'
const client = new ApolloClient({
cache: new InMemoryCache(),
link: new HttpLink({
uri: 'INSERT YOUR URL HERE',
useGETForQueries: true,
}),
})
export default client
query.ts – This will hold the queries that we want to make (The specific data that we want to fetch from the API). We will export the queries so that we can then use them in our components.
import { gql } from '@apollo/client'
const GET_PRODUCTS = gql`
query GetProducts {
products {
id
name
description
price
images {
id
url
fileName
width
height
}
}
}
`
export { GET_PRODUCTS }
Create a UI Component.
Loading the data is easy. The first thing we need to do is create a UI Component. You can use any number of ways to create a React component.
Wrap your React App in the Apollo provider and pass in the client we made earlier.
export default function App({ Component, pageProps }: AppProps) {
return (
<>
<ApolloProvider client={client}>
<GlobalStyle />
<Header />
<Component {...pageProps} />
</ApolloProvider>
</>
)
}
Now let’s create a custom hook so we can fetch the data.
useProducts.tsx – Here we import the query that we want and pass it into the useQuery hook from Apollo Client and then we return the states.
import { useQuery } from '@apollo/client'
import { GET_PRODUCTS } from '../../lib/graphql/query'
export const useProducts = () => {
const { loading, error, data } = useQuery(GET_PRODUCTS)
return { loading, error, data }
}
Finally, we need to use the hook in our component.
import { useProducts } from '../components/hooks/useProducts'
export default function Home() {
const { loading, error, data } = useProducts()
if (loading) return <p>Loading</p>
if (error) return <p>error</p>
return (
<ProductRows>
{!!data &&
data?.products.map((product: ProductType) => (
<Product
key={product.id}
id={product.id}
title={product.name}
price={product.price}
rating={4}
image={product.images[0].url}
/>
))}
</ProductRows>
)
}
Notice that we do not need a useState or useEffect to handle either the loading state, the error state or even the data. This is all returned and handled in the useProducts hook.
Conclusion
In the tutorial, you understood what is the GraphQL and how it is used to fetch data within your react application. In my opinion, it is the future and much easier and cleaner to fetch data this way.
You can watch the video of this explanation here.
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!