What are reducers in redux explain like i’m 5 years old?
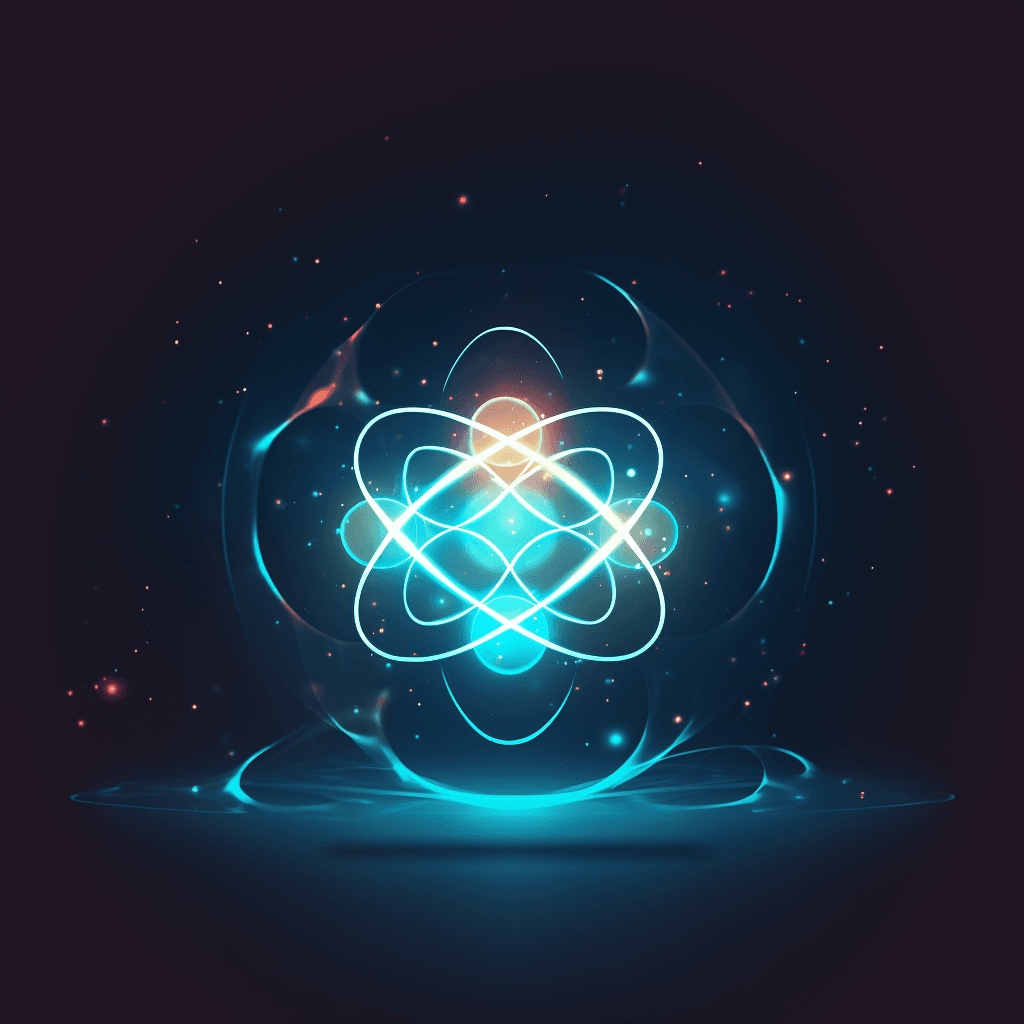
Reducers are special functions that help to manage information in a program called Redux. Think of Redux like a giant toy box with all sorts of toys inside. A reducer is like a special helper who takes out a toy, plays with it, and then puts it back in the toy box.
In Redux, there is something called “state” which is like a snapshot of what the toy box looks like at any given time. When we want to make a change to the toy box, we use something called an “action” which is like a set of instructions for the reducer to follow. The reducer takes these instructions and uses them to change the toy box by taking out some toys, playing with them, and then putting them back in the toy box in a different way.
import { configureStore } from "@reduxjs/toolkit";
import detailsReducer from "./reducers/detailsReducer";
import layerToggleReducer from "./reducers/layerToggleReducer";
import userReducer from "./reducers/userReducer";
export const store = configureStore({
reducer: {
user: userReducer,
layerToggle: layerToggleReducer,
details: detailsReducer,
},
});
export type RootState = ReturnType<typeof store.getState>;
export type AppDispatch = typeof store.dispatch;
So, a reducer is a special helper that takes instructions (actions) and uses them to change the toy box (state) in a specific way. And just like a real-life helper, a reducer can only change the toy box in certain ways, following the specific instructions it has been given.
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!