Understanding Deployment Strategies: Pros, Cons, and Implementation
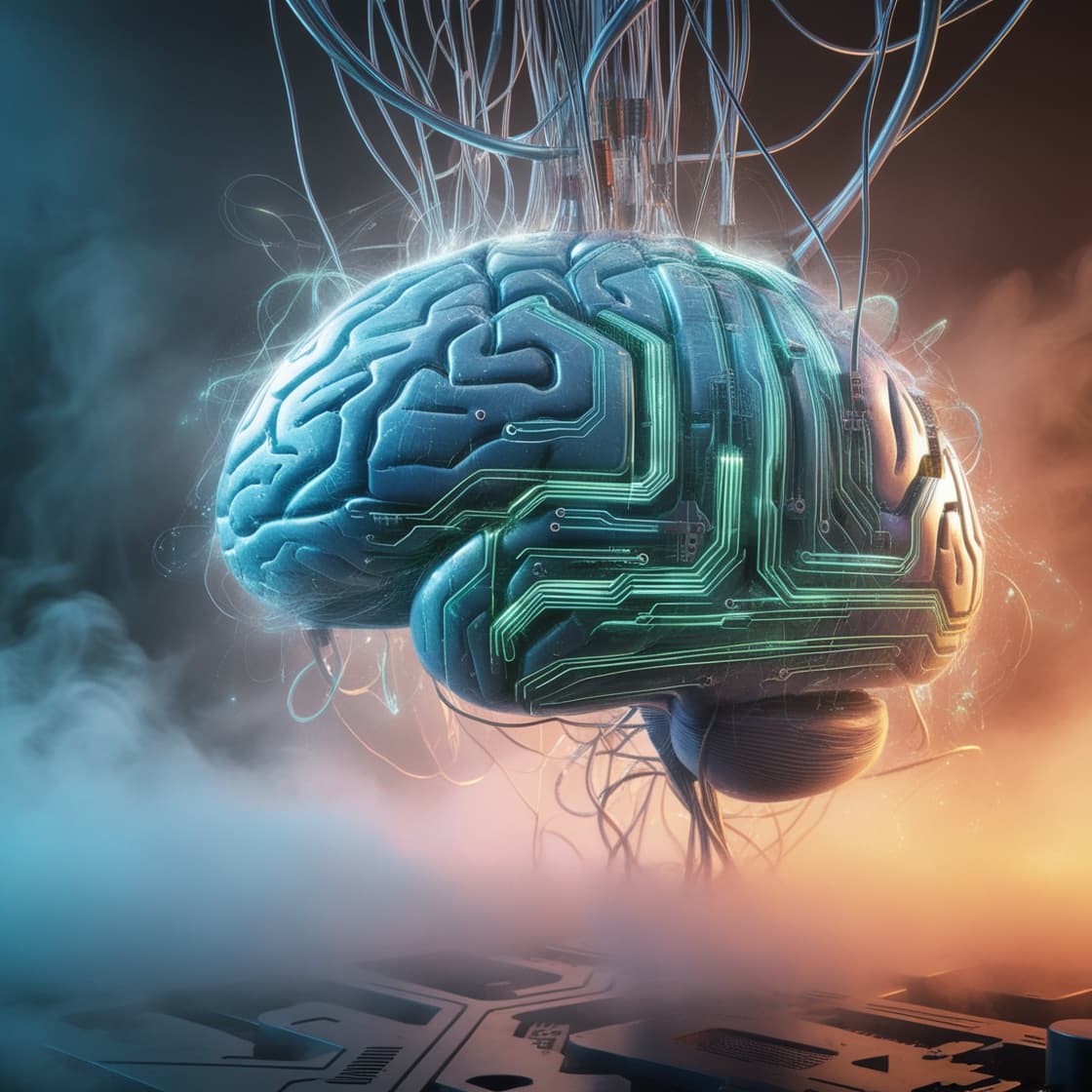
Deploying software is a critical phase in the software development lifecycle. The chosen deployment strategy can impact the stability, performance, and user experience of the application. This blog will explore various deployment strategies, discussing their advantages, disadvantages, and implementation details.
1. Recreate Deployment Strategy
Overview
The recreate strategy involves shutting down the old version of the application and then starting the new version. This approach is straightforward and easy to understand.
Pros
Simplicity: Easy to implement and understand. Resource Efficient: No need to run multiple versions simultaneously, conserving resources.
Cons
Downtime: There is inevitable downtime while the new version is being deployed. Risky: If the new version fails, the entire application is down.
Implementation
In Kubernetes, you can implement this strategy using the kubectl command:
kubectl rollout restart deployment/<deployment-name>
In Docker Swarm, the docker service update command can be used with the --update-parallelism 0 flag to stop the old version before starting the new one.
docker service update --image <new-image> --update-parallelism 0 <service-name>
2. Rolling Update Deployment Strategy
Overview
Rolling updates incrementally replace instances of the old version with instances of the new version. This ensures that the application remains available during the deployment.
Pros
Zero Downtime: Ensures the application is always available. Gradual Update: Allows monitoring and rollback if issues are detected.
Cons
Complexity: More complex to implement than the recreate strategy. Inconsistent State: Users may experience inconsistent behavior if interacting with different versions during the update.
Implementation
In Kubernetes, a rolling update is the default deployment strategy:
apiVersion: apps/v1
kind: Deployment
metadata:
name: example-deployment
spec:
replicas: 3
strategy:
type: RollingUpdate
template:
metadata:
labels:
app: example
spec:
containers:
- name: example-container
image: example-image:latest
In Docker Swarm, the docker service update command can be used without additional flags:
3. Blue-Green Deployment Strategy
Overview
Blue-green deployment involves running two identical production environments. The current version (blue) is live, while the new version (green) is deployed and tested. Once verified, traffic is switched from blue to green.
Pros
Zero Downtime: Seamless switch with no downtime. Rollback: Easy to revert to the previous version if needed. Testing: New version can be thoroughly tested before going live.
Cons
Resource Intensive: Requires double the resources to maintain two environments. Complexity: Managing two environments and switching traffic can be complex.
Implementation
This can be managed with a load balancer or DNS switch. For instance, in AWS, you can use Elastic Beanstalk to manage blue-green deployments:
- Deploy the new version to a new environment.
- Swap environment URLs.
In Kubernetes, you can manually handle this using services and deployments:
apiVersion: v1
kind: Service
metadata:
name: example-service
spec:
selector:
app: blue
ports:
- protocol: TCP
port: 80
targetPort: 8080
Switching traffic involves updating the service selector from app: blue to app: green.
4. Canary Deployment Strategy
Overview
Canary deployments involve releasing the new version to a small subset of users before rolling it out to the entire user base. This helps in identifying issues early without affecting all users.
Pros
Risk Mitigation: Limits exposure of new version issues. Gradual Rollout: Easier to monitor and control the deployment process.
Cons
Complexity: Requires additional setup for monitoring and traffic splitting. Delayed Feedback: Full user feedback takes longer.
Implementation
In Kubernetes, this can be managed with labels and multiple deployments:
apiVersion: apps/v1
kind: Deployment
metadata:
name: canary-deployment
spec:
replicas: 1
template:
metadata:
labels:
app: example
version: canary
spec:
containers:
- name: example-container
image: example-image:canary
A service then routes a percentage of traffic to the canary deployment using an ingress controller or service mesh like Istio.
5. A/B Testing Deployment Strategy
Overview
A/B testing is similar to canary deployments but focuses on testing different versions of the application (A and B) simultaneously to compare performance and user experience.
Pros
Data-Driven: Provides concrete data to compare different versions. User Feedback: Real user feedback helps in making informed decisions.
Cons
Complexity: Requires robust tracking and analysis tools. User Confusion: Users might get confused with different versions.
Implementation
A/B testing often uses feature flags or routing rules to direct users to different versions. In Kubernetes, you might use an ingress controller to route traffic based on headers or cookies.
6. Shadow Deployment Strategy
Overview
Shadow deployments route live traffic to the new version without affecting the response to the user. This allows testing in a real-world scenario without impacting users.
Pros
No Impact: Live traffic testing without affecting users. Real-World Testing: Identifies issues in real-world conditions.
Cons
Resource Intensive: Duplicates processing, requiring more resources. Complexity: Implementing shadow traffic routing and monitoring can be complex.
Implementation
In Kubernetes, this can be achieved using Istio or other service meshes to mirror traffic:
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: example-service
spec:
hosts:
- example.com
http:
- route:
- destination:
host: example-v1
mirror:
host: example-v2
Conclusion
Choosing the right deployment strategy depends on various factors such as application requirements, user base, available resources, and risk tolerance. Understanding the pros and cons of each strategy helps in making an informed decision that aligns with the project goals. Whether you prefer the simplicity of recreate deployments or the safety of canary releases, having a clear deployment plan is essential for the success of your software application.
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!