How to Fetch API Data in React Like a Pro
You've built a kickass React app and it's time to add some data. You could use an API, but what if you don't know how? Or maybe you've built an API with your favorite tool but have no idea how to fetch that data into your application? Don't worry — we're about to walk through the basics of fetching data from an API with React.
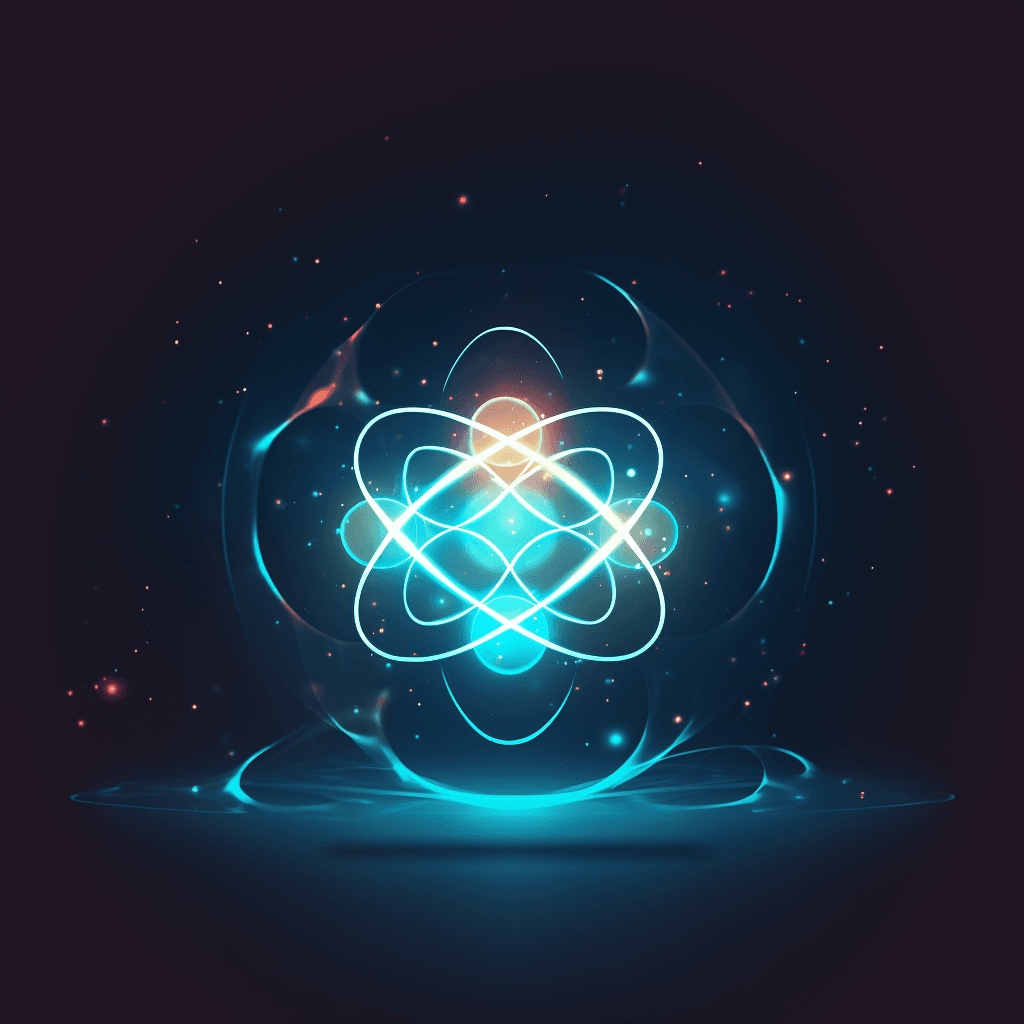
Fetch API data in React
You’re not limited to using the fetch API for getting data. You can also use it to create, read, update and delete (CRUD) data, which is what we’re going to look at next. You will need to know how to use this fetch API as a prerequisite. I recommend using the npm package axios which has tons of benefits.
How to fetch API Data with React Hooks
You'll need to install the following npm packages:
-
React (the base package for React) You can use Vite
-
useState (a simple state management utility)
-
useEffect (a lifecycle hook for managing side effects in React)
-
useReducer (a global state manager for React) - This is optional and can be managed with useState. An example of this is below
Refactor an API Call to Hooks in React
A React Hook is a function that lets you perform work before or after an API call.
There are three different types of Hooks:
-
useState()
, which is used for stateful components. You can use it to set up the initial state of an application, and also to update the state based on props or other external factors. -
useEffect()
, which is used for side effects (like using fetch). It runs once when the component mounts, then runs again if any props change mid-render cycle (like if we were using React Suspense). -
useCallback()
, which is used for asynchronous calls such as AJAX requests or delayed rendering data from local storage
Example in a real-world application
import React from 'react'
const FlightCard = () => {
const [isLoading, setIsLoading] = useState(true)
const [data, setData] = useState([])
useEffect(() => {
const url = 'https://jsonplaceholder.typicode.com/users'
// Allows us to intercept an API request so we can cancel anytime - sending signal in fetch will destroy immediately
const controller = new AbortController()
const { signal } = controller
const fetchData = async () => {
try {
const response = await fetch(url, { signal }).then((res) => res.json())
setData(response)
setIsLoading(false)
} catch (err) {
if (err instanceof Error) {
if (err.name === 'AbortError') {
console.log('api request has been cancelled')
}
console.log(err.name)
} else {
console.log('This is an unknown error')
}
}
}
fetchData()
return () => {
// cleanup the abort controller
controller.abort()
}
}, [])
if (isLoading) return <p>Loading...</p>
return (
<FlightCard title="Trending Destination">
{' '}
{data.length &&
data?.map((item) => (
<div key={item.id} className="destination">
{' '}
<li>{item.address.city}</li> <button>+</button>{' '}
</div>
))}{' '}
</FlightCard>
)
}
export default test
I have made a video on this that can be found on my Youtube Channel.
Conclusion
When you’re working with React and APIs, there are a lot of moving parts. This article covers everything from building an API call to wiring it up in your component lifecycle so that your app can fetch data without any hassle. I also showed how to make the most out of promises and hooks, so that you have all the tools at your disposal when it comes time to build something great!
See more blogs on my site also or check out videos on my Youtube Channel.