How is state Changed in redux explained like i’m 5 Years old?
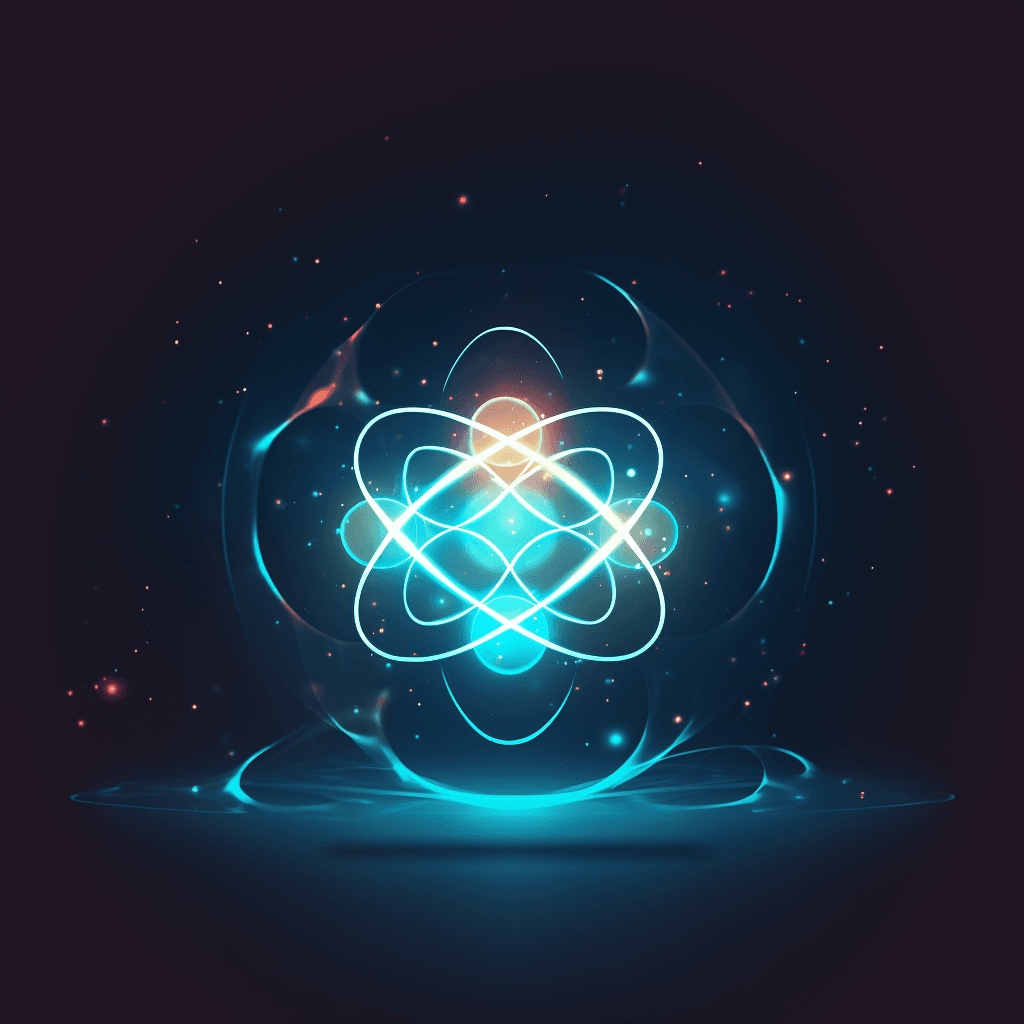
In Redux, “state” is like a box of toys that we want to change or update. But, we can’t just go into the box and start changing things, we have to follow some rules. Think of it like playing a game where you can only make moves by following certain instructions.
To change the state in Redux, we have to create something called an “action”. This is like a special set of instructions that tells Redux what we want to do with the toys in the box. We then give the action to something called a “reducer”, which is like a helper who knows how to follow the instructions in the action and make the changes to the toy box.
The reducer is like a cashier at a bank who can only do certain things with the money. In Redux, the reducer can only make changes to the state by following specific rules. It can’t just change the state however it wants to.
And, when we create a new state in Redux, we don’t actually change the old state. Instead, we create a whole new state by using the previous state and applying the changes from the action. This helps to keep things organized and easy to understand.
import { createSlice } from "@reduxjs/toolkit";
import type { PayloadAction } from "@reduxjs/toolkit";
import { RootState } from "../index";
import { UserType } from "../../../types/store";
const initialState: UserType = {
user: null,
};
export const userSlice = createSlice({
name: "user",
initialState,
reducers: {
signInToAccount: (state, action: PayloadAction<any>) => {
state.user = action.payload;
},
signOutFromAccount: (state) => {
state.user = null;
},
},
});
export const { signInToAccount, signOutFromAccount } = userSlice.actions;
export const selectUser = (state: RootState) => state.user.user;
export default userSlice.reducer;
// We will change the state of the user from null to the string below
dispatch(signInToAccount('Imran Codes'))
So, in summary, we change the state in Redux by creating an action that tells Redux what we want to do with the toys in the box, and then giving the action to a reducer who knows how to make the changes. And when we make a new state, we don’t actually change the old state, we just create a whole new one.
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!