Dynamic Imports To Stop Server-Side Rendering in NextJS
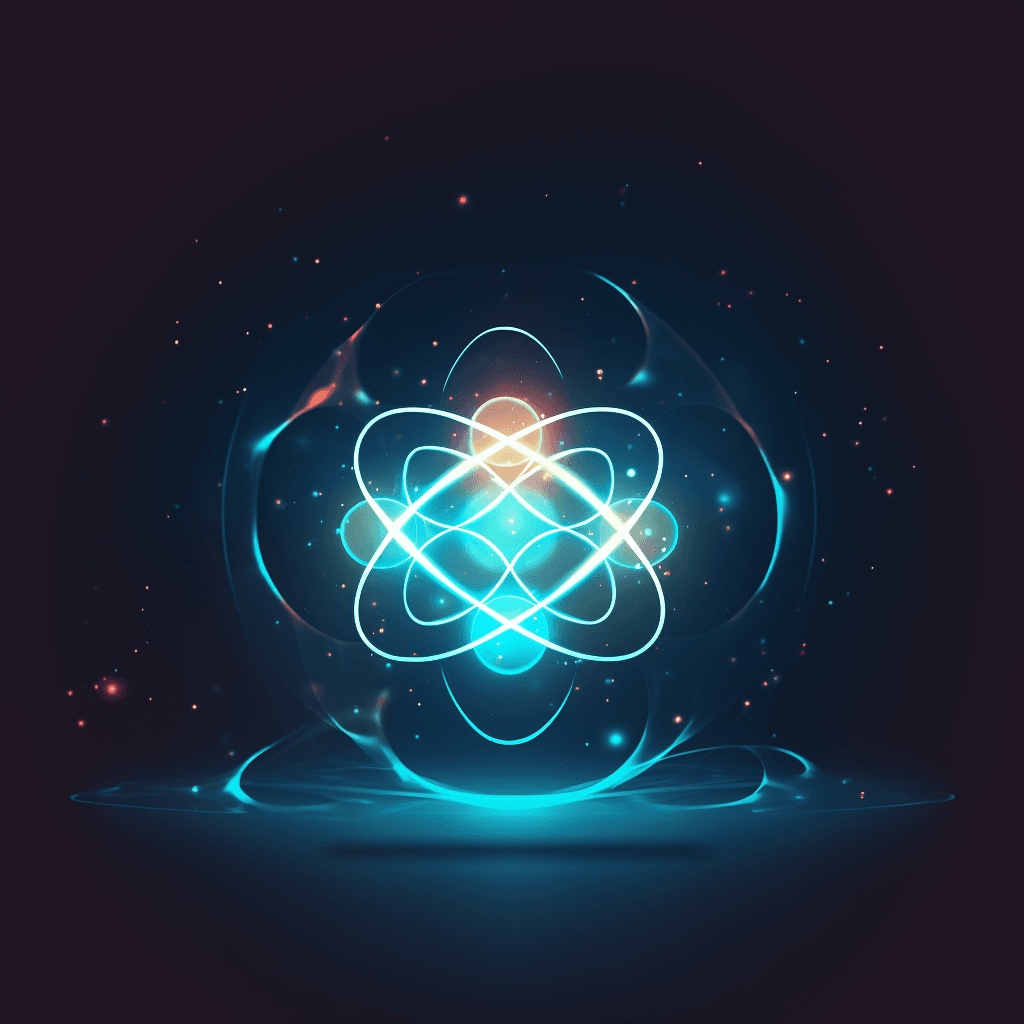
Have you ever seen this error “ReferenceError: window is not defined”:
NextJS is a framework for building server-rendered web apps, but it’s sometimes necessary to use dynamic imports for local state management or data fetching. This can lead to some strange behavior where the server-rendered app doesn’t render correctly due to the presence of an import() statement. Next.JS will try to generate the HTML of the page server side for better performance and SEO.
What do we mean by dynamic?
Dynamic imports are the act of importing a module at runtime. When we use third-party npm packages sometimes they only work in the client whereas we can use SSR (server-side rending) in NextJS which is why we need dynamic imports.
By dynamic, we mean the following:
The import statement is not evaluated at compile-time (CoffeeScript’s require function or Babel’s require plugins).
The import statement is evaluated at runtime and may include data that could not have been known by the compiler.
Can I use async await?
You can use async-await to stop server-side rendering.
Async await is a JavaScript language feature that makes asynchronous code look similar to synchronous code because it allows you to write asynchronous functions in the same way as if they were synchronous.
NextJS uses the next-async package for asynchronous code, which includes async and await keywords. These keywords are used to write asynchronous functions using sequential execution flow (just like how you would write synchronous functions).
How is this possible?
While there are a number of ways to load modules dynamically in NextJS, this is the simplest method.
To use dynamic imports in NextJS, you must first import dynamic from next into your server-side entry point:
import React from "react";
import dynamic from "next/dynamic";
import Head from "next/head";
const MapContainer = dynamic(
() => import("react-leaflet").then((mod) => mod.MapContainer),
{ ssr: false }
);
const TileLayer = dynamic(
() => import("react-leaflet").then((mod) => mod.TileLayer),
{ ssr: false }
);
const Marker = dynamic(
() => import("react-leaflet").then((mod) => mod.Marker),
{ ssr: false }
);
const Popup = dynamic(() => import("react-leaflet").then((mod) => mod.Popup),
{ ssr: false });
Conclusion
I hope this article helped demystify dynamic imports and how they can be used to stop server-side rendering in NextJS. If you’re looking for more information, check out my video on this for further detail, and check out the Imran Codes Youtube Channel also.