Building a Progressive Web App with React and Next.js in 2023
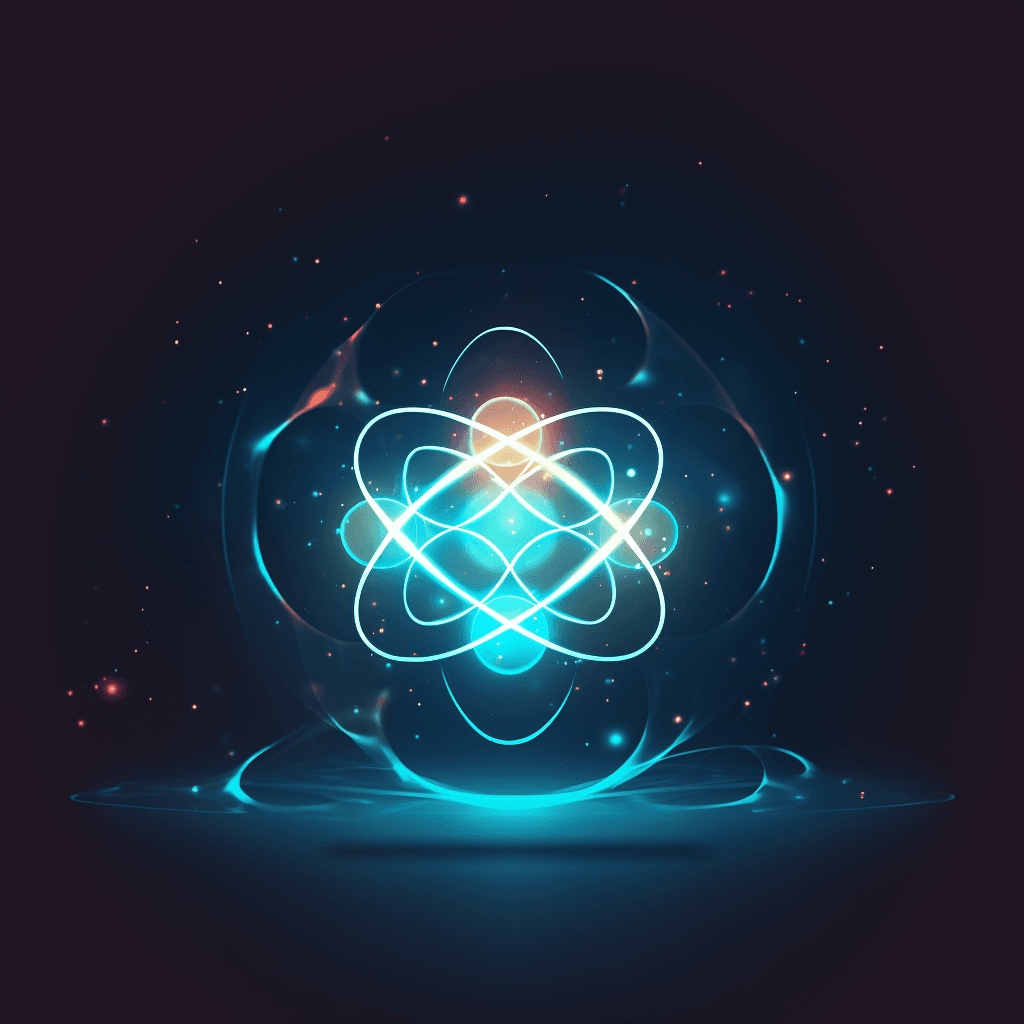
Welcome! In this post, I will be building a progressive web application (PWA) using React and Next.js. I will explain the concepts of PWAs, service workers, and offline caching. I’ll start by talking about what a PWA is and why you should build one. Then I’ll go through how to install the necessary tools on your machine as well as some basic setup on GitHub. Finally, I’ll walk through creating an app with React and Next.js that uses Google’s Firebase Cloud Functions for authentication purposes!
What are Progressive Web Apps (PWAs)?
Progressive web apps (PWAs) are an exciting new way for developers to create fast, reliable and engaging web experiences. They combine the best of the web and mobile experiences to give users a smooth experience regardless of their network connection or device.
PWAs are built using modern web technologies like service workers, which enable them to work offline; Web App Manifests that allow you to specify which icons should be displayed in different locations on your home screen; App Shell Model which allows us to pre-load content before it’s needed so that pages load faster when they’re first accessed by users; Progressive Enhancement so our PWA will still work even if JavaScript is disabled in their browser or they have no internet connection at all!
Why use React?
The first step in building a progressive web app is to choose your technology stack. There are many frameworks available, but as always I will be using React because it’s easy to learn and has a large community of developers.
Next, I’ll set up our project with Next.js–a framework that allows us to write server-side code in JavaScript while automatically generating HTML files for each request.
The Setup
- Install the dependencies.
- Create a new project.
- Install Next.js and React:
- Next: npm install next –save-dev
- React: npm install react react-dom –save-dev
The Testing App
To get started, you need to import the testing app. This will be your starting point for creating your own progressive web application (PWA). Before we do that though, let’s set up our testing environment by creating an npm package and installing some dependencies:
npm init -y
// Next, install NextJS and React:
yarn add next react react-dom@next react-router-dom@next react-scripts@1.1.7 --dev
// Next, create a directory for your project called pwa-demo: mkdir pwa-demo && cd $_
Lets create a manifest.json file:
{
"name": "Imran Codes",
"short_name": "Imran",
"theme_color": "#fff",
"background_color": "#000",
"display": "standalone",
"scope": "/",
"start_url": "https://imrancodes.com/",
"description": "The ultimate destination for anyone looking to learn how
to code and become a web development expert!",
"orientation": "any",
"icons": [
{
"src": "https://imrancodes.com/imran.jpg",
"sizes": "1024x1024"
}
]
}
Implementing a Service Worker:
-
Service workers are a key technology behind PWAs. They act as a proxy between the web app, the network, and the browser.
-
Service workers enable offline functionality, background synchronization, and caching of assets, making PWAs usable even when the network connection is unreliable or unavailable.
In a React and Next.js project, you can create a service worker file (e.g., service-worker.js) to customize the caching and offline behavior.
Here’s an example of a basic service worker implementation in a React and Next.js project:
// service-worker.js
self.addEventListener('install', event => {
event.waitUntil(
caches.open('my-cache').then(cache => {
return cache.addAll([
'/',
'/index.html',
'/styles.css',
'/script.js',
'/image.jpg'
// Add more assets to cache as needed
]);
})
);
});
self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request).then(response => {
return response || fetch(event.request);
})
);
});
The above code registers the service worker and caches essential assets during the installation phase. When a fetch event occurs (e.g., loading a page or requesting a resource), the service worker intercepts it and checks if the requested resource is available in the cache. If so, it responds with the cached version; otherwise, it fetches the resource from the network.
Note: If you use create-react-app then all of the above is preinstalled and you can just tweak it.
Takeaway:
- PWAs are web apps that combine the best of the web and mobile apps, such as push notifications and offline support. They’re fast, reliable, and engaging.
- React is a JavaScript library for building user interfaces that has become popular in recent years because it makes it easier to write code that’s reusable between projects. It also provides a great developer experience with tools like hot module reloading and state restoration baked right into your editor of choice!
- Next is an opinionated framework for creating PWAs using React along with many other features like routing out-of-the box so you don’t have to worry about those details while learning how everything else works together at once!
Conclusion
In this post, I’ve discussed how to create a progressive web app using React and Next.js. I started with an overview of the technologies that make up PWAs, then went into detail about what they mean for your site’s users. I also showed you how to set up your development environment so that you can start building your own PWA today!
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!