Best Practices for Testing React and Next.js Applications in 2023
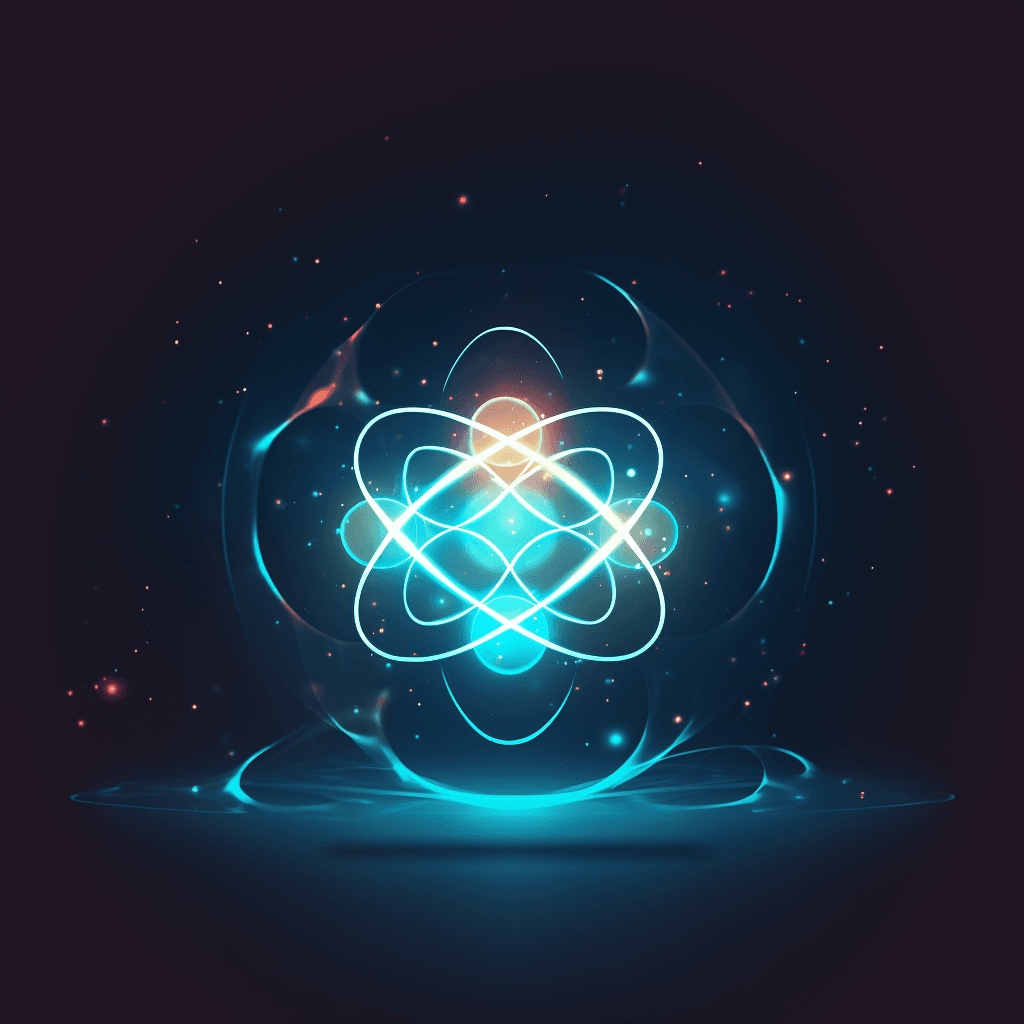
Testing is an essential part of any software project, but tests are often overlooked when it comes to React and Next.js. In this article, I’ll cover the main types of testing that you can do with your React and Next.js codebases so that you can ship high-quality code with confidence.
Unit testing
Unit testing is the process of isolating and testing individual units of source code. Unit tests are written by developers as part of their development process, usually after having written new code or changed existing code. Unit tests can be run repeatedly, without requiring human intervention (for example, via a build script).
The purpose of unit testing is to ensure that individual pieces of software work as expected and continue to do so over time as new requirements or bugs are introduced into the system.
Unit testing is one of the first steps in the development process. It’s usually done after coding dependant on your preference of course, but before integration testing and system testing. Unit tests are used to ensure that each part of your application works as expected.
When it comes to testing React and Next.js applications in 2023, there are several effective strategies and tools available to ensure the quality and reliability of your codebase. Here are some best practices along with relevant coding examples:
Unit testing involves testing individual components or functions in isolation to verify their correctness. You can use testing frameworks like Jest[1], which is commonly used with React and Next.js. Jest provides powerful assertion utilities, test runners, and mocking capabilities. Here’s an example of a unit test for a React component using Jest:
// Example test file: MyComponent.test.js
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders the component correctly', () => {
render(<MyComponent />);
const headingElement = screen.getByText('Hello, World!');
expect(headingElement).toBeInTheDocument();
});
Integration testing
Integration testing is a type of software testing that verifies the interaction between components in an application. It is performed after unit testing, as a way to verify that the components work as expected when used together.
Integration tests can be done manually or automated with tools like Selenium, Cypress, TestCafe and others.
In this article we will explore some best practices for integration testing React applications using TestCafe (a popular open-source tool).
We will also go over some of the common pitfalls you might encounter when writing integration tests.
In this article, we’ll go over some of the best practices for integration testing React applications using TestCafe (a popular open-source tool) . We will also go over some of the common pitfalls you might encounter when writing integration tests.
- Integration Testing: Integration testing involves testing how different components and modules interact with each other. React Testing Library[1] is a popular choice for integration testing in React and Next.js applications. It provides a set of utilities to render components and simulate user interactions. Here’s an example of an integration test using React Testing Library:
// Example test file: Integration.test.js
import { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders the component and interacts with it', () => {
render(<MyComponent />);
const buttonElement = screen.getByRole('button');
expect(buttonElement).toBeInTheDocument();
// Simulate a user click
fireEvent.click(buttonElement);
const messageElement = screen.getByText('Button clicked!');
expect(messageElement).toBeInTheDocument();
});
End-to-end testing
End-to-end testing, also known as integration testing, is the most expensive form of testing. It involves running your entire application in a real browser using automated tests that mimic user interactions. Because it requires a lot of upfront investment and time to set up, end-to-end tests are usually only used for high-value applications and large codebases.
However, they’re also the most reliable type of test because they simulate real-world usage scenarios and can catch bugs that other types of automated unit or integration tests wouldn’t find–especially when dealing with complex user interfaces.
- End-to-End (E2E) Testing: E2E testing involves testing the entire application flow from start to finish, including user interactions, API calls, and UI rendering. For Next.js applications, Cypress[1] is a popular E2E testing framework. It provides a clean syntax and allows you to write expressive tests. Here’s an example of an E2E test using Cypress:
// Example Cypress test file: e2e.spec.js
describe('My Next.js App', () => {
it('successfully loads the homepage', () => {
cy.visit('/');
cy.contains('Welcome to My Next.js App').should('be.visible');
});
it('allows the user to perform a search', () => {
cy.visit('/');
cy.get('input[name="search"]').type('Hello');
cy.contains('Search').click();
cy.url().should('include', '/search');
cy.contains('Search Results').should('be.visible');
});
});
The best way to ensure the quality of your React and Next.js codebase is by testing it.
Testing helps you answer questions like: “Is my code working?” or “Does this new feature break something else?” Testing also helps you improve the quality of your code by helping you identify bugs before they become problems for users.
Testing is not just for developers; everyone on a team should be involved in testing their own work as well as other parts of the system that they use or touch at any given time. This includes designers and product managers who are responsible for making sure that their work has been implemented correctly, QA specialists who test new features before they’re released into production (and beyond), developers who write unit tests to make sure their changes haven’t broken anything else in the system–the list goes on!
End-to-end tests are also the best way to ensure that your application works correctly when deployed in production. This is because they run outside of your development environment, so there’s no chance that a local bug could affect the results.
Conclusion
I hope that this article has helped you get started with testing your React and Next.js applications. Good luck!
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!