ReactJS for beginners
ReactJS is a JavaScript library created by Facebook for building user interfaces. It is used for building large applications that can be rendered on both the client and server sides. ReactJS uses a "virtual DOM", which allows it to be more efficient than other libraries. It has features like data binding and modularity, which make it easier to maintain large applications than other libraries.
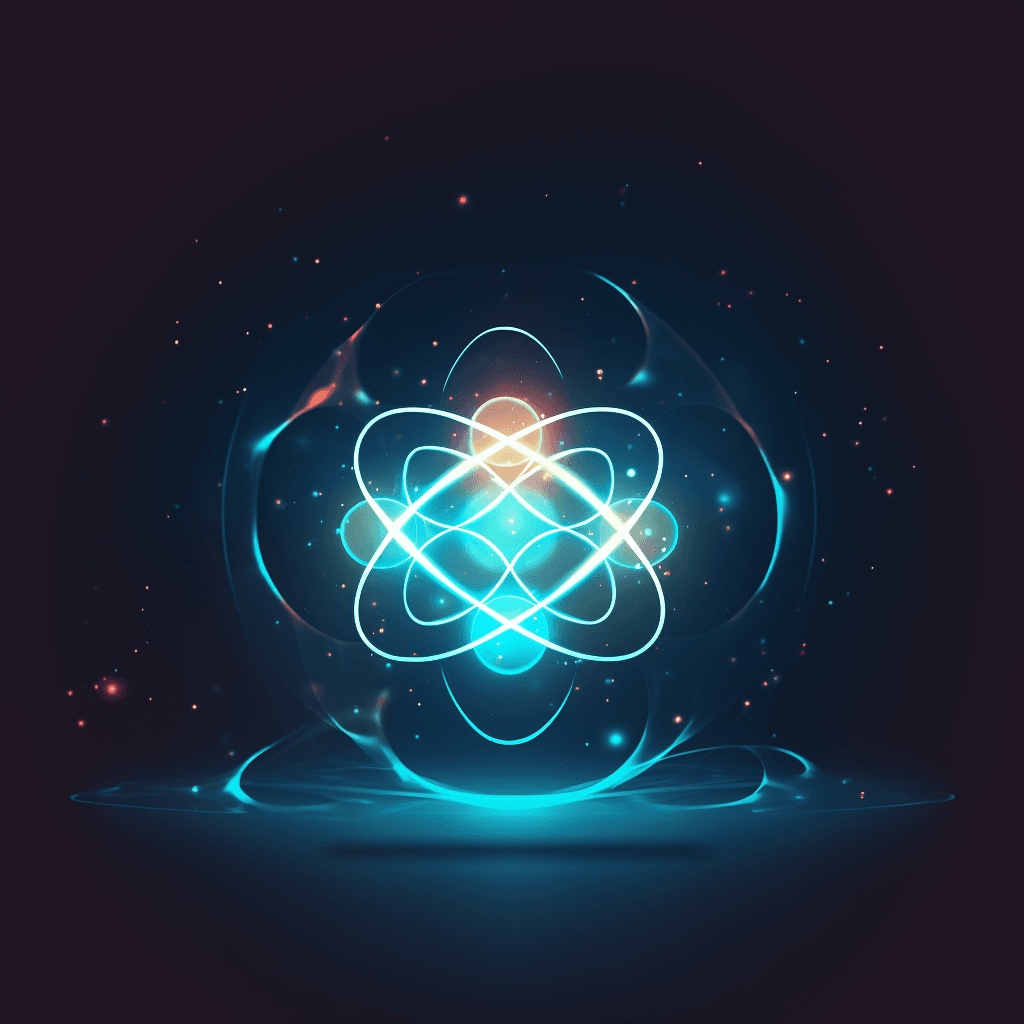
What is ReactJS?
React is a JavaScript library for building user interfaces. React makes it painless to create interactive UIs. Design simple views for each state in your application, and React will efficiently update and render just the right components when your data changes. Declarative views make your code more predictable and easier to debug.
When to use ReactJS?
React is a great solution for building user interfaces. It's the best choice when you need to build large applications that are complex and reusable. React is also good for web and mobile applications, including hybrid apps.
What makes React special? Its one-way data flow helps you implement clean code with pure functions, so you can write your components in a declarative way by describing what the component should look like instead of how it should behave. This makes React intuitive and easy to use, even for beginners!
What are the advantages of ReactJS?
React is a view library, which means that it helps you build user interfaces. It's also a JavaScript library, which means it can be used with any programming language that supports JavaScript. React is open source and available on GitHub. In addition to being an open-source library, React has some other advantages as well:
-
React is easy to use: The main reason for its popularity among developers is its simplicity; if you're new to building web apps or have been building them for years and want to try something new, learning React will be easy because all you need is basic knowledge of HTML and CSS.
-
You can use it with other libraries: If your company uses another JavaScript library like AngularJS (or just Angular) or VueJS along with several others then there are no issues since both these frameworks are compatible with each other as well as having their own set of advantages over each other
It's easy to learn: React is very easy to use, and if you're new to building web apps or have been building them for years and want to try something new, then learning React will be easy because all you need is basic knowledge of HTML and CSS.
What is JSX?
JSX is a JavaScript syntax extension. It allows you to write HTML-like code in JavaScript files, and it's compiled into JavaScript by Babel.
You can use JSX as an alternative to creating React components with createClass and extends . That makes sense because the JSX syntax looks like HTML, but it's actually more than that: JSX lets you express your data structures with plain JavaScript! This way, when the time comes for React to render your component’s user interface, all of those data structures are ready and waiting for React so that it can render them into DOM nodes on the fly while keeping everything up-to-date on each change.
List some of the key features of React.
React is an open-source JavaScript library for building user interfaces. It's fast and efficient, allowing you to create high-quality and interactive user experiences that are smooth, dynamic, and engaging. Here are some of the key features of React:
-
JSX: JSX is a JavaScript syntax extension that allows you to write HTML-like tags in your code. The tags get compiled into real DOM elements when you run the application. This makes it easier to develop applications as you can write out your UI using familiar HTML tags instead of learning yet another markup language like XML or JSON.
-
Virtual DOM: The Virtual DOM concept was introduced by React developers in order to improve performance by avoiding unnecessary updates made by other frameworks such as AngularJS which use a dirty checking mechanism where they compare all changes with the previous state on every update or render call which can have a significant impact on performance if there are a large number of components being updated at once. In contrast, virtual DOM uses a diffing algorithm that works by comparing the previous version with the current one only after deciding whether there has been any change between them (if yes then rendering will take place).
In the UI for example if you try to change the text of an element and then revert back to the original one. In such cases, we can use the force update method provided by React which forces React to compare both versions of the virtual DOM and update the real DOM accordingly.
What is a component in ReactJS?
A component is a piece of UI. It's built up from smaller parts and can be reused throughout your application.
There are two major types of components: React Components and Custom Elements. React Components use JSX to create an element that's part of your application's tree structure, whereas Custom Elements are regular HTML elements with their own JavaScript API (e.g., ).
Creating a new component is easy! Here's an example of a button component using ES6 syntax:
import React from 'react'
function Button() {
return (
<div>Button</div>
)
}
export default Button
What are the differences between HTML and JSX?
JSX is JavaScript and HTML mixed together. It is not parsed by the browser, but instead by Babel. This allows us to use syntax that can't be parsed at runtime (e.g., className) directly in our JavaScript files.
The biggest difference between JSX and HTML is that JSX is not like normal HTML; it's pure JavaScript. This means that we cannot simply take an HTML file and write it as a component with JSX—we need to translate certain elements' attributes into React components.
How does state differ from props in react?
In React, the state is a property of a component. The props are passed from parent to child components and are immutable. In other words, you can't change a prop after it's been passed down from parent to child. On the other hand, the state is mutable and can be changed internally by a component. Props are only used for passing data between components at runtime but not for changing it at run time.
Conclusion
React is a great tool for building user interfaces. It allows you to build reusable components that can be integrated into your application with ease. React is a powerful library that works well with any other front-end framework. In this article, we went over some of the most common questions asked during interviews related to ReactJS and how you can prepare for them by practicing on Imran Codes Youtube Channel!