How to add navigation in my react application
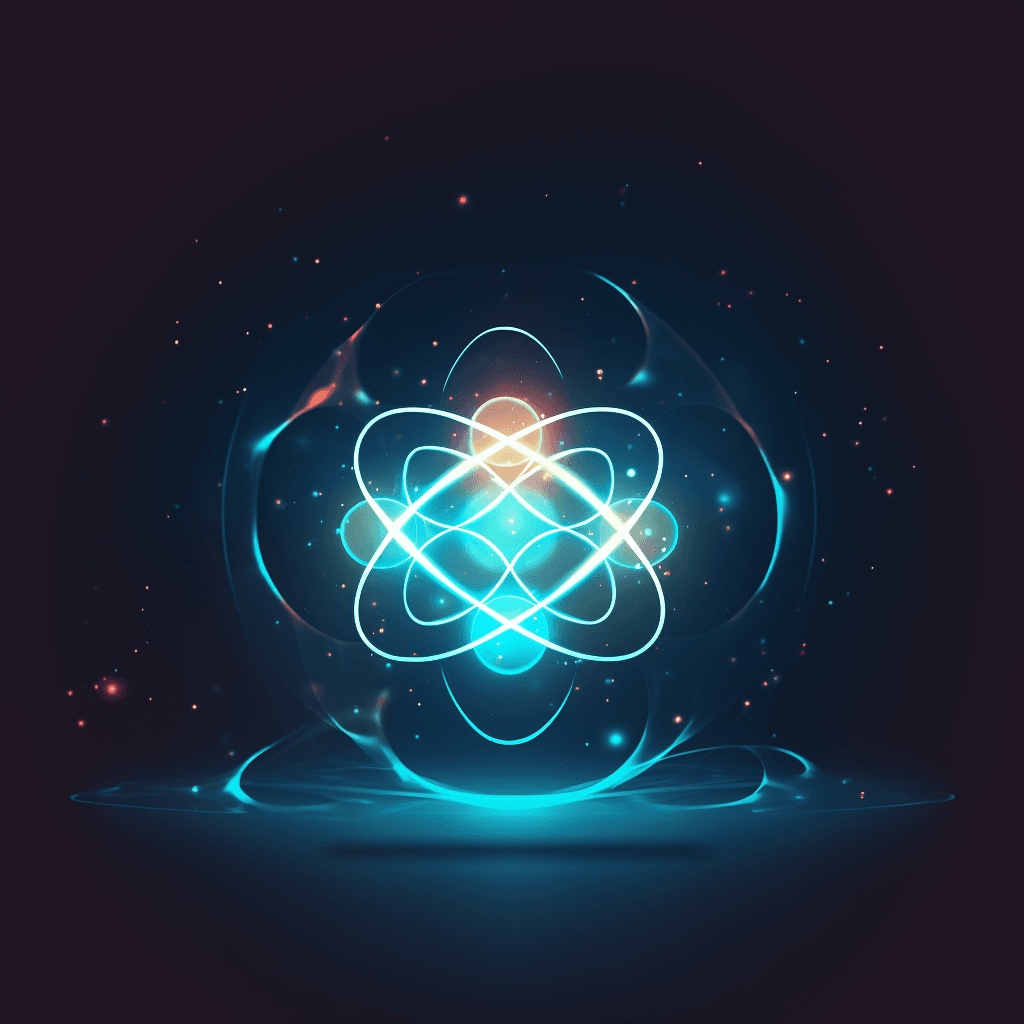
To add navigation to your React application you need to follow a few steps:
So, what is React Router?
It's a collection of navigational components that compose declaratively with your application. It allows you to define your application's navigation in a single place and render it wherever you need it.
Now install react-router-dom by running the following command.
To install it, run:
npm install react-router-dom
or
yarn add react-router-dom
Next, we have to import the necessary components from the react-router-dom package in the index.js file.
The next step is to import the necessary components from the react-router-dom package in the index.js file. You can see below:
import React from 'react'
import ReactDOM from 'react-dom/client'
import './index.css'
import App from './App'
import { BrowserRouter } from 'react-router-dom'
import Navbar from './components/Navbar'
const root = ReactDOM.createRoot(document.getElementById('root'))
root.render(
<React.StrictMode>
<BrowserRouter>
<Navbar />
<App />
</BrowserRouter>
</React.StrictMode>
)
As you can see above we have a BrowserRouter that wraps our App and Nav bar component. The Nav bar component will be shown on all page and we then define our routes in App.js below:
import React from 'react'
import { Route, Routes } from 'react-router-dom'
import Card from './components/Card'
import Create from './components/Create'
import Destinations from './components/Destinations'
import Details from './components/Details'
import NotFound from './components/NotFound'
function App() {
return (
<Routes>
<Route path="/create" element={<Create />} />
<Route path="/hotels/:id" element={<Details />} />
<Route path="*" element={<NotFound />} />
<Route path="/" element={<Card />} />
<Route path="/destinations" element={<Destinations />} />
</Routes>
)
}
export default App
Routes.
The two main arguments required for a route are the path and the element that will show with that route. The rule of thumb is:
Add a route to the App component:
Create a new route with a path of '/create'.
Add the component you want to render in the element
Think about how you will modularize your app and how you will use react-router for it.
In order to think about how you will modularize your app and how you will use react-router for it, there are some things that need to be done before adding any code.
First, make sure that you have a good folder structure for your application. It's important to have everything organized in a way that makes sense. For example, if I were building an app where users can upload photos and then view them later on (like Instagram or Facebook), I might create these folders:
+src/
-
actions are functions that both fetch data and send back new data on each request (think of them as middleware)
-
components hold up the UI like buttons, forms, etc...
-
common code such as stylesheets or utils should go here because they're used by more than one file/page/feature of our application
Conclusion
I hope this article will help you to create navigation in your react application.
In conclusion, I would like to say that you should think about modularizing your app and how you will use react-router for it, before starting with actual coding I hope the examples have helped. To see the example code in action, watch my Youtube video on this.
See more blogs on my site also or check out videos on my Youtube Channel.