Elevating Frontend Development: A Guide to CI/CD Pipelines with GitHub Actions
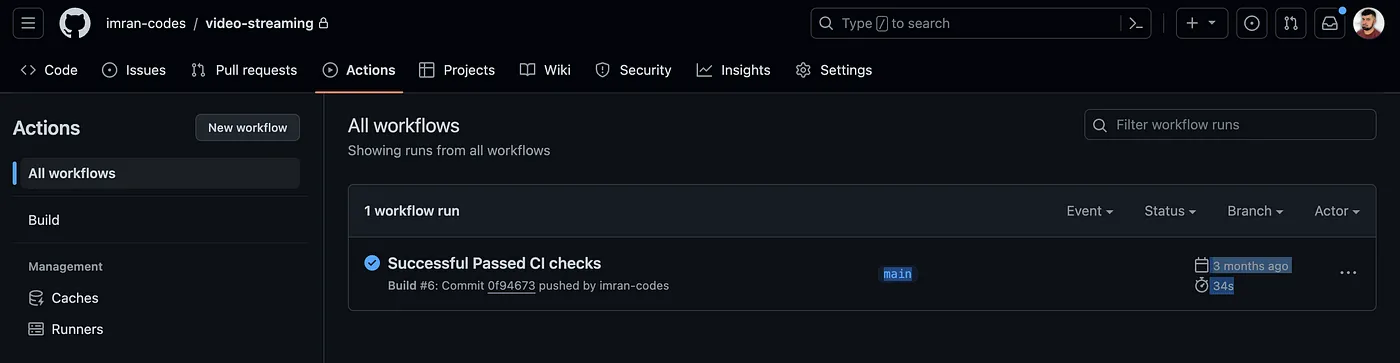
Introduction:
Frontend development has evolved rapidly over the years, and with the growing complexity of web applications, maintaining a high standard of code quality has become more crucial than ever. Continuous Integration (CI) and Continuous Deployment (CD) pipelines play a pivotal role in achieving this by automating the process of testing, linting, and deploying code changes. In this article, we’ll delve into the world of CI/CD pipelines, focusing on how GitHub Actions can empower frontend developers to ensure code quality before merging changes.
Understanding CI/CD Pipelines:
Continuous Integration (CI): CI involves regularly merging code changes from multiple contributors into a shared repository. The primary goal is to detect and address integration issues early in the development process. Automated builds, tests, and code analysis are key components of a CI pipeline.
Continuous Deployment (CD): CD, an extension of CI, takes the automation a step further by deploying changes to a staging or production environment after successful CI. It ensures that your application is always in a deployable state.
Setting Up GitHub Actions for Frontend Projects: GitHub Actions is a powerful CI/CD solution provided by GitHub, allowing you to define workflows using YAML configuration files. Let’s start by creating a basic workflow for a frontend project.
Example GitHub Actions Workflow (.github/workflows/main.yml):
name: Frontend CI/CD
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout Repository
uses: actions/checkout@v2
- name: Set Up Node.js
uses: actions/setup-node@v3
with:
node-version: [16.x, 18.x]
- name: Install Dependencies
run: npm install
- name: Linting
run: npm run lint
- name: Unit Tests
run: npm test
- name: Build
run: npm run build
- name: Deploy to Staging
if: github.event_name == 'push' && github.ref == 'refs/heads/main'
run: |
# Add deployment script or commands here
In this example, the workflow is triggered on each push to the main branch. It performs linting, unit testing, and builds the project. Additionally, it includes a deployment step (to staging in this case), which you can customize based on your deployment strategy.
Integrating Linting and Testing:
Linting and testing are crucial for maintaining code quality. Let’s explore how to integrate these processes into your GitHub Actions workflow.
Linting with ESLint:
Install ESLint:
npm install eslint --save-dev
Create an ESLint configuration file (.eslintrc.js):
module.exports = {
extends: 'eslint:recommended',
rules: {
// Your custom linting rules go here
},
env: {
browser: true,
node: true,
},
};
Update your GitHub Actions workflow:
# ... (previous steps)
- name: Linting
run: npx eslint .
Testing with Jest:
Install Jest:
npm install jest --save-dev
Create a Jest configuration file (jest.config.js):
module.exports = {
testEnvironment: 'jsdom',
// Additional Jest configuration goes here
};
Update your GitHub Actions workflow:
# ... (previous steps)
- name: Unit Tests
run: npx jest
By integrating ESLint for linting and Jest for testing, you’re ensuring that your code adheres to coding standards and functions as expected.
Conclusion
Implementing CI/CD pipelines with GitHub Actions empowers frontend developers to maintain a high code quality standard. By automating linting and testing processes, you catch issues early, fostering a more collaborative and efficient development environment. Take the first step towards a streamlined development workflow, and watch your frontend projects flourish with increased reliability and maintainability.
Remember, this is just a starting point. Tailor the workflows to your project’s specific needs and gradually introduce more advanced deployment strategies as your team and application grow. Happy coding!
See more blogs on my site also or check out videos on the Imran Codes Youtube Channel!